Understanding and Implementing a Basic Roulette Game in Python
Roulette, a classic game of chance found in casinos worldwide, offers a blend of excitement and anticipation that few other games can match. The game involves a spinning wheel, a small ball, and a table of bets. In this article, we will dive into the basics of roulette and how you can implement a simple version of this game in Python.
The Essence of Roulette
At its core, roulette is a game that revolves around a wheel marked with numbers ranging from 0 to 36, with each number being uniquely colored, either red or black (commonly, the number 0 is represented in green). The game is orchestrated by a dealer who initiates the action by spinning the wheel and releasing a small ball into it. Players participating in the game place their bets based on their predictions of where the ball will eventually come to rest.
Roulette offers a variety of betting options, adding an element of excitement and strategy to the game. Players can place their bets on a specific number, wager on whether the outcome will be an odd or even number, or predict the color in which the ball will land, among other available options. These diverse betting possibilities contribute to the allure of roulette, making it a popular choice among casino enthusiasts worldwide.
Mathematical Formulas and Probabilities in Roulette: Understanding the Odds
Certainly, here are some mathematical formulas related to roulette:
Probability of Hitting a Specific Number (Single Number Bet):
- Probability = 1/37 for European Roulette (with one zero)
- Probability = 1/38 for American Roulette (with two zeros)
Probability of Winning an Even Money Bet (e.g., Red/Black, Odd/Even):
- Probability = 18/37 for European Roulette (excluding the zero)
- Probability = 18/38 for American Roulette (excluding both zeros)
Expected Value (EV) of a Bet:
- EV = (Probability of Winning * Potential Payout if You Win) – (Probability of Losing * Amount Bet)
Standard Deviation of Bets:
- Standard Deviation = √[N * (P * (1-P)) * (1 – (Potential Payout if You Win / Amount Bet)^2)]
where N is the number of bets, P is the probability of winning, and the rest of the variables are as defined in the formula.
House Edge (for a Specific Bet):
- House Edge = 100% – (Probability of Winning * Potential Payout if You Win) – (Probability of Losing * Amount Bet)
These formulas are used by players and casinos to calculate various probabilities and expected values associated with different bets in roulette. They help players make informed decisions about their bets and assist casinos in setting their odds to maintain a house edge.
Implementing Roulette in Python
Python, known for its simplicity and readability, is an ideal language for simulating games like roulette. Here’s a step-by-step guide to implementing a basic version:
- Setting Up the Wheel: Our Python version uses a list to represent the roulette wheel, with numbers ranging from 0 to 36.
- Placing Bets: Players can input their bet type – choosing a specific number, odd, or even.
- Spinning the Wheel: The
random
module selects a random number, simulating the spinning of the roulette wheel. - Determining the Outcome: The program compares the bet with the outcome and declares whether the player wins or loses.
This implementation is a basic model and can be expanded with additional features like betting on red or black, implementing a betting system, or adding a graphical user interface.
Basic Roulette Game Simulation in Python
Here’s a simple Python code snippet that simulates a basic roulette game, where a player places a bet on a specific number, and the program determines whether the player wins or loses. Please note that this is a basic simulation and does not represent a real casino game.
import random
def roulette_game():
# Generate a random winning number (0 to 36 for European Roulette)
winning_number = random.randint(0, 36)
# Player places a bet on a specific number (you can change this)
player_bet = 17
# Determine if the player wins or loses
if player_bet == winning_number:
return "Congratulations! You won! The winning number is {}.".format(winning_number)
else:
return "Sorry, you lost. The winning number is {}.".format(winning_number)
# Run the roulette game
result = roulette_game()
print(result)
In this code, we simulate a roulette game by generating a random winning number and having the player place a bet on a specific number (in this case, 17). The program then checks if the player’s bet matches the winning number and provides a corresponding message.
Keep in mind that this is a simplified example for demonstration purposes, and real roulette games are far more complex and involve various types of bets and payouts.
Python Code Example
Here’s a snippet of the Python code for a simple roule tte game:
import random
def play_roulette():
numbers = list(range(0, 37))
bet_type = input("Type of bet (number, even, odd): ").lower()
# ... additional code ...
play_roulette()
This code sets up the game, takes the user’s bet, spins the wheel, and announces the outcome.
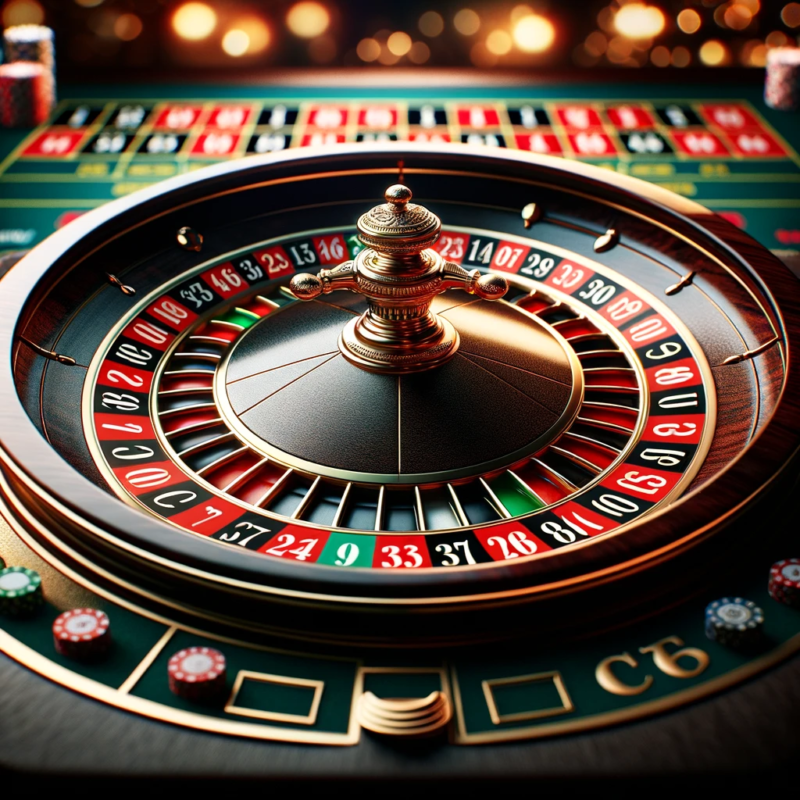
The Educational Value of Simulating Games
Programming a game like roulette in Python is not just about the game itself. It’s an excellent way to learn programming concepts and problem-solving skills. By simulating games, one can understand the application of random number generation, user input handling, and basic game logic in programming.
Analyzing and Simulating Roulette Scenarios with Python
Certainly, here are a couple of problems related to roul ette that can be solved using Python:
Problem 1: Calculate the Expected Value of a Bet
In roulette, you want to calculate the expected value (EV) of a specific bet. The EV helps you determine whether a bet is favorable or not in the long run. For example, you can calculate the EV of a bet on a specific number or an even-money bet like red/black.
Solution:
You can create a Python function that calculates the EV of a given bet based on the probabilities and payouts associated with that bet. Here’s a basic example:
def calculate_ev(probability_win, payout_win, probability_lose):
ev = (probability_win * payout_win) - (probability_lose * 1)
return ev
# Example: Calculate EV for a bet on Red in European Roulette
probability_red_win = 18/37 # Probability of winning on red
payout_red_win = 2 # Payout for winning on red (double the bet)
probability_red_lose = 19/37 # Probability of losing
ev_red = calculate_ev(probability_red_win, payout_red_win, probability_red_lose)
print("Expected Value (EV) for a bet on Red:", ev_red)
Problem 2: Simulate Multiple Roulette Spins
You want to simulate multiple spins of a roul ette wheel and track the outcomes. This can help you analyze different betting strategies or study the long-term behavior of the game.
Solution:
You can use Python to simulate roulette spins and record the results over a specified number of spins. Here’s a basic example:
import random
def simulate_roulette_spins(num_spins):
results = []
for _ in range(num_spins):
winning_number = random.randint(0, 36)
results.append(winning_number)
return results
# Example: Simulate 100 roulette spins
num_spins = 100
spin_results = simulate_roulette_spins(num_spins)
print("Results of {} roulette spins:".format(num_spins))
print(spin_results)
These problems and solutions demonstrate how Python can be used to analyze and simulate roulette-related scenarios. You can extend these examples for more complex analyses or to explore various betting strategies.
Conclusion
While our Python roulette game is a basic representation, it captures the essence of the real-world game. It’s a perfect starting point for beginners to dive into the world of programming and game development. Whether you’re a novice coder or an experienced programmer, creating games like roulette can be both fun and educational.
